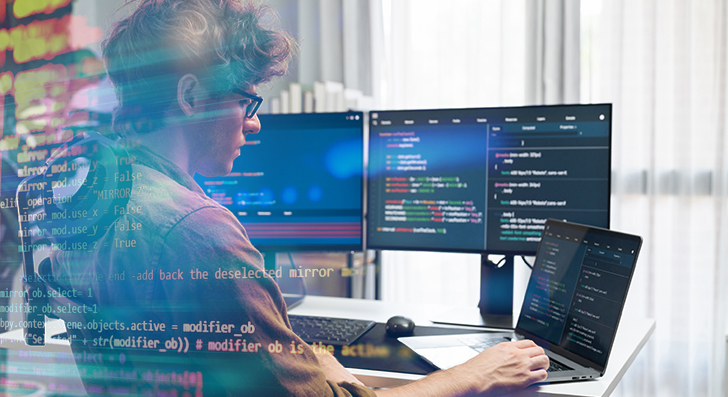
Scalability signifies your software can cope with progress—a lot more users, extra knowledge, and a lot more site visitors—without breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not a thing you bolt on later—it ought to be component within your program from the start. Several purposes fail if they develop rapid since the first style can’t cope with the extra load. Like a developer, you might want to Feel early regarding how your system will behave under pressure.
Get started by creating your architecture being flexible. Stay away from monolithic codebases where by every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial pieces. Every module or provider can scale By itself without affecting The entire technique.
Also, give thought to your database from day a single. Will it need to have to take care of one million users or perhaps a hundred? Select the appropriate style—relational or NoSQL—based on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important point is to prevent hardcoding assumptions. Don’t create code that only operates beneath recent problems. Contemplate what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-pushed units. These assistance your application cope with additional requests devoid of receiving overloaded.
If you Construct with scalability in mind, you're not just making ready for fulfillment—you happen to be cutting down long run complications. A effectively-planned system is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the right databases is actually a important part of making scalable programs. Not all databases are constructed the same, and utilizing the Improper you can sluggish you down or perhaps induce failures as your app grows.
Start by being familiar with your knowledge. Is it remarkably structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great match. These are sturdy with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with additional site visitors and details.
Should your data is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and may scale horizontally extra effortlessly.
Also, look at your read and generate patterns. Will you be doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases which will handle higher publish throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of avoidable joins. Normalize or denormalize your data based on your access patterns. And usually keep track of database efficiency as you develop.
In brief, the correct database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Just take time to choose properly—it’ll conserve lots of trouble afterwards.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, every small hold off provides up. Badly created code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct efficient logic from the beginning.
Start off by composing clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward a single works. Keep the features short, centered, and easy to check. Use profiling equipment to find bottlenecks—destinations in which your code takes far too lengthy to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These frequently gradual issues down much more than the code itself. Ensure that Each and every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and rather find certain fields. Use indexes to hurry up lookups. And steer clear of executing a lot of joins, Specifically throughout large tables.
Should you see exactly the same knowledge being requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database operations any time you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and Developers blog helps make your app additional economical.
Remember to test with huge datasets. Code and queries that operate high-quality with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers plus more traffic. If everything goes through one server, it'll quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming website traffic throughout a number of servers. As opposed to a single server performing all the do the job, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it with the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for fast entry.
2. Customer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching minimizes databases load, improves pace, and will make your app additional effective.
Use caching for things which don’t change typically. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but impressive resources. Jointly, they help your app cope with much more end users, continue to be quick, and Recuperate from challenges. If you propose to develop, you require both of those.
Use Cloud and Container Resources
To create scalable apps, you would like resources that allow your app improve conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to buy hardware or guess long term capability. When site visitors will increase, it is possible to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, with no surprises. Docker is the most well-liked tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your application into companies. You are able to update or scale pieces independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments implies you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease chance, and help you remain centered on building, not fixing.
Watch Everything
Should you don’t watch your software, you won’t know when items go Completely wrong. Monitoring assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These show you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how often problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you deal with difficulties rapidly, typically just before customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you are able to roll it again ahead of it triggers real destruction.
As your app grows, visitors and details enhance. Without having checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, monitoring will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you could Develop applications that grow easily devoid of breaking under pressure. Start off compact, Believe massive, and Establish intelligent.